Tutorial: Creating an Endpoint to Download Files using Golang and Gin-Gonic
Tutorial: Creating an Endpoint to Download Files using Golang and Gin-Gonic
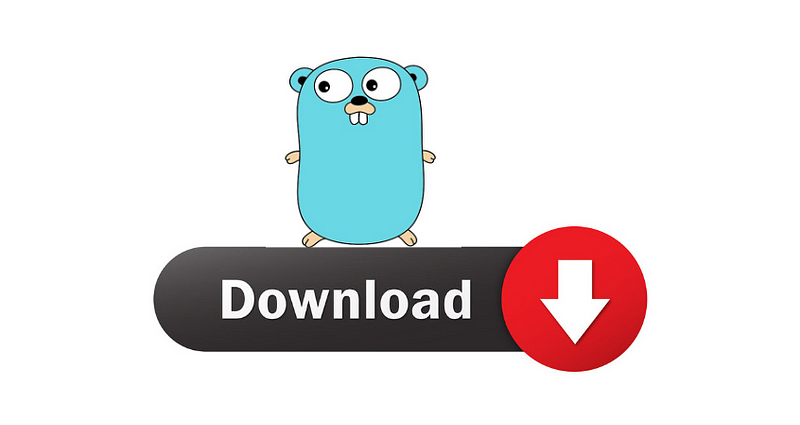
In this tutorial, you will learn how to create an endpoint on a Go web server using the Gin-Gonic framework that allows users to download files, whether it’s a PDF file or an MP3 music file. You will discover how to configure routes to enable file downloads and how to handle HTTP headers properly to facilitate content download. Throughout this tutorial, two examples will be provided: one for downloading a PDF file and another for downloading an MP3 music file.
Prerequisites:
- Have Go (Golang) installed on your system.
- Install Gin-Gonic by running the following command:
go get github.com/gin-gonic/gin
.
Step 1: Create a Project
The first thing you need to do is create a Go project and set up a Gin-Gonic web server. Below is a base code example for your project:
package main
import (
"github.com/gin-gonic/gin"
"net/http"
"os"
)
func main() {
r := gin.Default()
// Routes for downloading files
// Here you will add your routes for downloading PDF and music
r.Run(":8080") // Start the server on port 8080
}
Step 2: Create a Route to Download a PDF File
Let’s create a route that allows users to download a PDF file.
// Route for downloading the PDF file
r.GET("/download-pdf", func(c *gin.Context) {
// Define the location of the PDF file on the server
filename := "path/to/file.pdf" // Change this path to where your PDF file is located
// Check if the file exists
_, err := os.Stat(filename)
if err != nil {
c.JSON(http.StatusNotFound, gin.H{
"error": "File not found",
})
return
}
// Set headers for download
c.Header("Content-Description", "File Transfer")
c.Header("Content-Transfer-Encoding", "binary")
c.Header("Content-Disposition", "attachment; filename="+filename)
c.Header("Content-Type", "application/pdf")
c.Header("Content-Length", "0")
// Send the file as a response
c.File(filename)
})
Step 3: Create a Route to Download a Music File (MP3)
Next, we’ll create a route to allow the download of an MP3 music file.
// Route for downloading the music file
r.GET("/download-music", func(c *gin.Context) {
// Define the location of the music file on the server
filename := "path/to/file.mp3" // Change this path to where your music file is located
// Check if the file exists
_, err := os.Stat(filename)
if err != nil {
c.JSON(http.StatusNotFound, gin.H{
"error": "File not found",
})
return
}
// Set headers for download
c.Header("Content-Description", "File Transfer")
c.Header("Content-Transfer-Encoding", "binary")
c.Header("Content-Disposition", "attachment; filename="+filename)
c.Header("Content-Type", "audio/mpeg") // Adjust the MIME type according to your music file format
c.Header("Content-Length", "0")
// Send the file as a response
c.File(filename)
})
Step 4: Test Your Server
Now that you’ve set up routes for downloading PDF and music files, you can start your Gin-Gonic server by running the program. Access the routes you’ve defined for file downloads and verify that they work correctly.
go run main.go
Download the PDF file
curl -o file.pdf http://localhost:8080/download-pdf
curl -o file.pdf
: This command will download the file and save it locally with the name "file.pdf".http://localhost:8080/download-pdf
: This is the URL of the route for downloading the PDF file.
The response will be the download of the PDF file to your local directory with the name “file.pdf”.
Download the music (mp3) File
curl -o music.mp3 http://localhost:8080/download-music
curl -o music.mp3
: This command will download the music file and save it locally with the name "music.mp3".http://localhost:8080/download-music
: This is the URL of the route for downloading the MP3 music file.
The response will be the download of the music file to your local directory with the name “music.mp3”.
Conclusion
In this tutorial, you’ve learned how to create a Go web server using the Gin-Gonic framework and set up routes to allow file downloads, whether it’s a PDF file or an MP3 music file. This functionality is useful for providing users with the ability to download content from your web application. Be sure to adjust file paths and locations according to your specific needs. Now you can easily provide your users with the capability to download files efficiently.