The Importance of Implementing SOLID Principles in Software Projects: Foundations for Excellence in Development
The Importance of Implementing SOLID Principles in Software Projects: Foundations for Excellence in Development
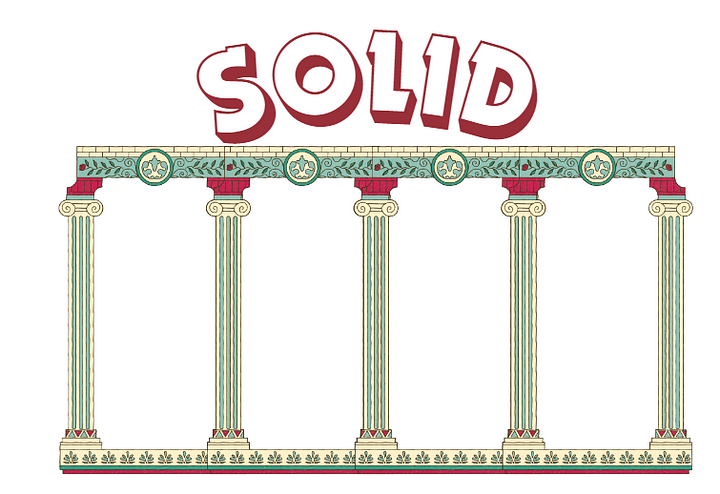
Software development is a continuously evolving field, driven by the need to create more robust, maintainable, and scalable applications. In this context, the SOLID principles stand out as a fundamental guide to achieving excellence in software design and programming. In this article, we will explore in-depth the importance of implementing SOLID principles in software projects and how they can transform the quality and longevity of our applications.
What Are the SOLID Principles?
SOLID is an acronym that represents five software design principles formulated by Robert C. Martin, an expert in software development and a renowned author. Each of these principles focuses on specific aspects of software design with the goal of producing clean, maintainable, and extensible code. Here is a brief overview of each of the five SOLID principles.
1. Single Responsibility Principle (SRP)
This principle states that a class should have only one reason to change. In other words, each class should have a specific responsibility and should not have more than one reason to be modified. This promotes modularity and prevents the creation of overloaded classes with multiple functions.
2. Open/Closed Principle (OCP)
The OCP focuses on the extensibility of software. It asserts that software entities (classes, modules, etc.) should be open for extension but closed for modification. This means that new functionalities can be added without changing existing code, promoting stability.
3. Liskov Substitution Principle (LSP)
The LSP pertains to inheritance and states that objects of a subclass should be able to replace objects of the base class without affecting program integrity. This ensures a consistent relationship between classes and prevents unexpected outcomes.
4. Interface Segregation Principle (ISP)
The ISP advocates for creating specific and cohesive interfaces instead of general ones. Classes should not be forced to implement methods they do not use. This prevents the creation of classes with excessive unwanted functions.
5. Dependency Inversion Principle (DIP)
The DIP suggests that high-level classes should not depend on low-level classes but both should depend on abstractions. This promotes flexibility and eases component substitution without altering high-level code.
The Importance of SOLID in Software Development
In the ever-evolving landscape of software development, the quest for creating robust, maintainable, and adaptable applications remains a top priority. Within this pursuit, the SOLID principles have emerged as a beacon guiding developers towards excellence in software design and coding. In this article, we’ll delve into the significance of implementing SOLID principles in software development and explore how they fundamentally transform the quality and longevity of our applications.
Maintainability
Implementing SOLID principles facilitates breaking down a system into smaller, manageable components. This simplifies error identification and correction, as well as the introduction of new features without fearing unwanted side effects. The application of SRP and OCP, in particular, contributes to more maintainable code by reducing complexity and constraining modification points.
Scalability
SOLID principles promote a design that efficiently adapts to future changes and expansions. The OCP allows for extension without modification, which is crucial for scaling applications as requirements change and functionalities grow.
Clarity and Understanding
Adhering to these principles leads to cleaner and more organized code. Classes with a single responsibility (SRP) and a cohesive interface (ISP) are easier to comprehend and maintain. This benefits development teams by facilitating collaboration and reducing the learning curve for new team members.
Code Reusability
The DIP encourages the creation of abstractions and dependency inversion. This eases the reuse of components in different parts of the system or even in future projects. Code complying with these principles becomes a valuable library of reusable resources.
Error Reduction
The clarity and modularity promoted by SOLID reduce the likelihood of introducing errors into the code. The LSP and ISP, in particular, ensure that classes and interfaces are consistent and predictable, contributing to software quality.
Reduction of Maintenance Costs
Adhering to SOLID principles reduces the need for frequent code modifications and costly repairs, saving resources throughout the software’s lifecycle.
Facilitates Debugging
Code following SOLID principles is easier to debug since issues tend to be confined to specific modules rather than spreading throughout the system.
Simplifies Documentation
A coherent and modular design simplifies the creation of effective documentation, benefiting developers and support teams.
Enhances Collaboration
Larger development teams can collaborate more effectively when they follow solid design principles, as the code is more predictable and less prone to unexpected errors.
Promotes Good Development Practices
SOLID principles encourage good development practices such as using design patterns and writing unit tests, resulting in higher-quality code.
Adopts Object-Oriented Design (OOP)
SOLID closely aligns with object-oriented design principles, improving code consistency and facilitating the adoption of OOP.
Speeds up Development
Despite the initial perception that SOLID might slow down development, its proper implementation can, in the long run, speed up the process by minimizing obstacles and errors.
Prepares for Changing Requirements
With a SOLID-based design, changes in project requirements are more manageable and less disruptive.
Improves Communication
Adherence to solid principles improves communication among team members by setting clear expectations for code structure.
Competitive Advantage
Applications developed with SOLID principles tend to be more reliable and quick to adapt to changing market demands, providing a competitive edge for businesses.
Practical Example of SOLID
Consider a simple example in the context of an employee management system. We will apply the SRP, which dictates that a class should have a single responsibility.
Suppose we have a class called Employee
that has two responsibilities: calculating salaries and sending payment notifications. The class can be divided into two separate classes: SalaryCalculator
and PaymentNotifier
, each with a single responsibility.
package main
import (
"fmt"
)
// Employee struct represents an employee.
type Employee struct {
Name string
Salary float64
}
// SalaryCalculator calculates the salary for an employee.
type SalaryCalculator struct{}
func (sc SalaryCalculator) CalculateSalary(employee Employee) float64 {
// Salary calculation logic
return employee.Salary
}
// PaymentNotifier sends payment notifications to employees.
type PaymentNotifier struct{}
func (pn PaymentNotifier) SendPaymentNotification(employee Employee) {
// Payment notification sending logic
fmt.Printf("Payment notification sent to %s\n", employee.Name)
}
func main() {
employee := Employee{Name: "John Doe", Salary: 5000.0}
salaryCalculator := SalaryCalculator{}
paymentNotifier := PaymentNotifier{}
salary := salaryCalculator.CalculateSalary(employee)
fmt.Printf("%s's salary is $%.2f\n", employee.Name, salary)
paymentNotifier.SendPaymentNotification(employee)
}
This refactoring improves code maintainability and clarity by adhering to the Single Responsibility Principle.
Conclusion
The importance of implementing SOLID principles in software projects is undeniable. These principles offer a structured approach to software design that results in cleaner, maintainable, and scalable code. Adherence to SOLID not only enhances software quality but also facilitates collaboration among development teams and reduces maintenance costs throughout the application's lifecycle. Ultimately, SOLID is a fundamental pillar for achieving excellence in software development and successfully addressing the challenges of the ever-evolving digital world.